The Top 5 Data Structures Every Developer Should Master
In the ever-evolving world of software development, understanding the core data structures is crucial for writing efficient and scalable code. This article delves into the top five data structures that every developer should master, highlighting their importance and applications, to get placed into a big MNC Companies, and to perform effectively in Coding Interviews. By mastering these fundamental structures, developers can optimize their code, improve performance, and tackle complex problems with ease. Whether you're a seasoned programmer or just starting out, these data structures will form the backbone of your coding toolkit. Let's explore how these essential tools can revolutionize your development process.
Top 5 Data Structures
1. Graphs
Many difficult problems that are asked in Coding interviews use graphs. A graph is a non-linear data structure that consists of a set of nodes, known as vertices, and the connections between them, called edges1. Graphs are incredibly versatile and can represent various real-world systems and relationships.
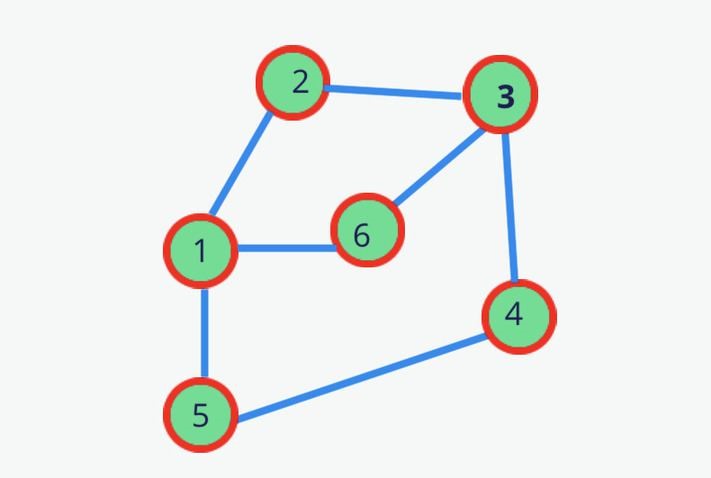
Graph Example
If the edges of a graph have a direction, then it is directed graph otherwise it is undirected graph.
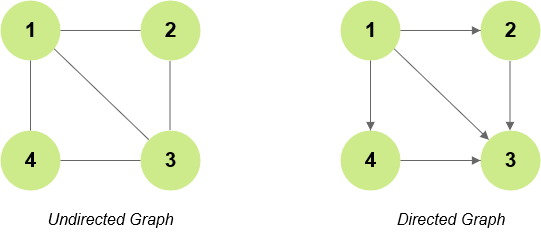
Graph Example
When to use Graphs and its Applications?
Graphs are incredibly useful for solving problems that involve relationships and connections between entities. Here are some applications:
Examples:
Pathfinding and Navigation(Finding shortest path between two loacations etc.)
Search engines like Google use graphs to rank web pages based on their link structure.
Platforms like Netflix and Amazon use graphs to suggest products or content based on user interactions and preferences.
and a lot more.
Important graph concepts
Here are few graph related concepts that you should read about:
-
Graph Search
Some famous graph search algorithms that you should read about are
- Breadth First Search
- Depth First Search
- Dijkstra's Algorithm
-
Topological Sort
-
Loop in a graph
Note: Will cover these topics in later articles.
2. Stack & Queues
Stack
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed. Think of it like a stack of plates: you can only take the top plate off the stack, and you can only add a new plate on top. A Stack is aalso used in recursion to store function calls.
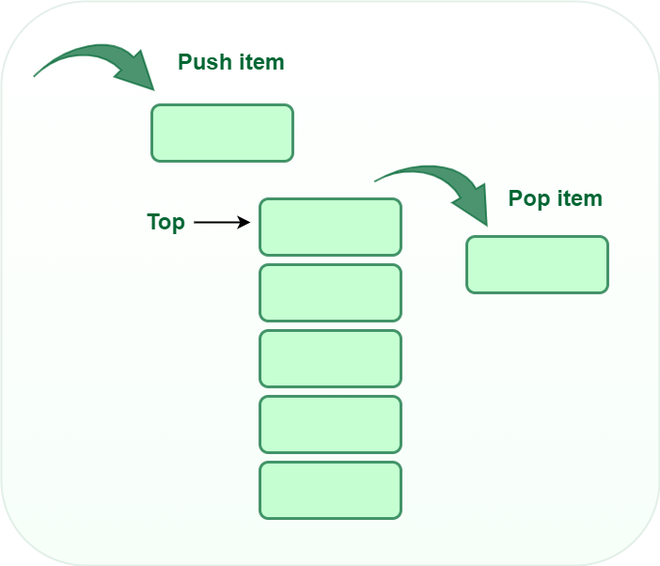
Stack Example
How elements are stored:
Push Operation: Adds an element to the top of the stack.
Pop Operation: Removes the element from the top of the stack.
Top/Peek Operation: Returns the top element without removing it.
Queue
A queue is a linear data structure that the First In, First Out (FIFO) principle. This means that the first element added to the queue will be the first one to be removed. Imagine a line of people waiting for a bus: the first person in line is the first to get on the bus.
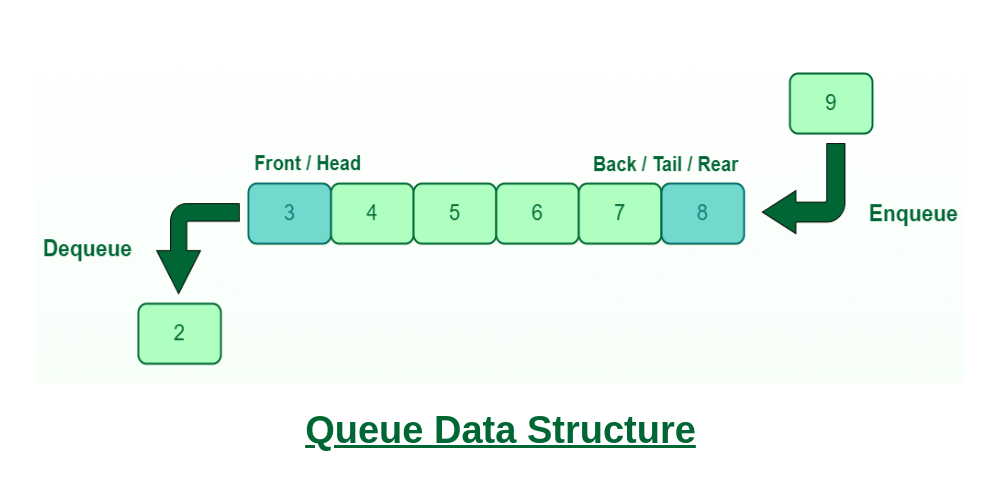
Queue Example
How elements are stored:
Enqueue Operation: Adds an element to the end (rear) of the queue.
Dequeue Operation: Removes the element from the front of the queue.
Front Operation: Returns the front element without removing it.
Rear Operation: Returns the last element without removing it.
3. HashMap/HashTable
HashMaps and HashTables are key data structures for fast key-value data retrieval, using hash functions to map keys to values. These structures are efficient, typically performing operations in constant time, O(1), making them vital for tasks like caching, database indexing, and network routing. Key concepts include the load factor, which measures the ratio of elements to array size, and rehashing, which resizes the table when the load factor is too high. Using immutable keys prevents changes in hash codes, ensuring data integrity. Mastering these structures is crucial for writing efficient, high-performance code.
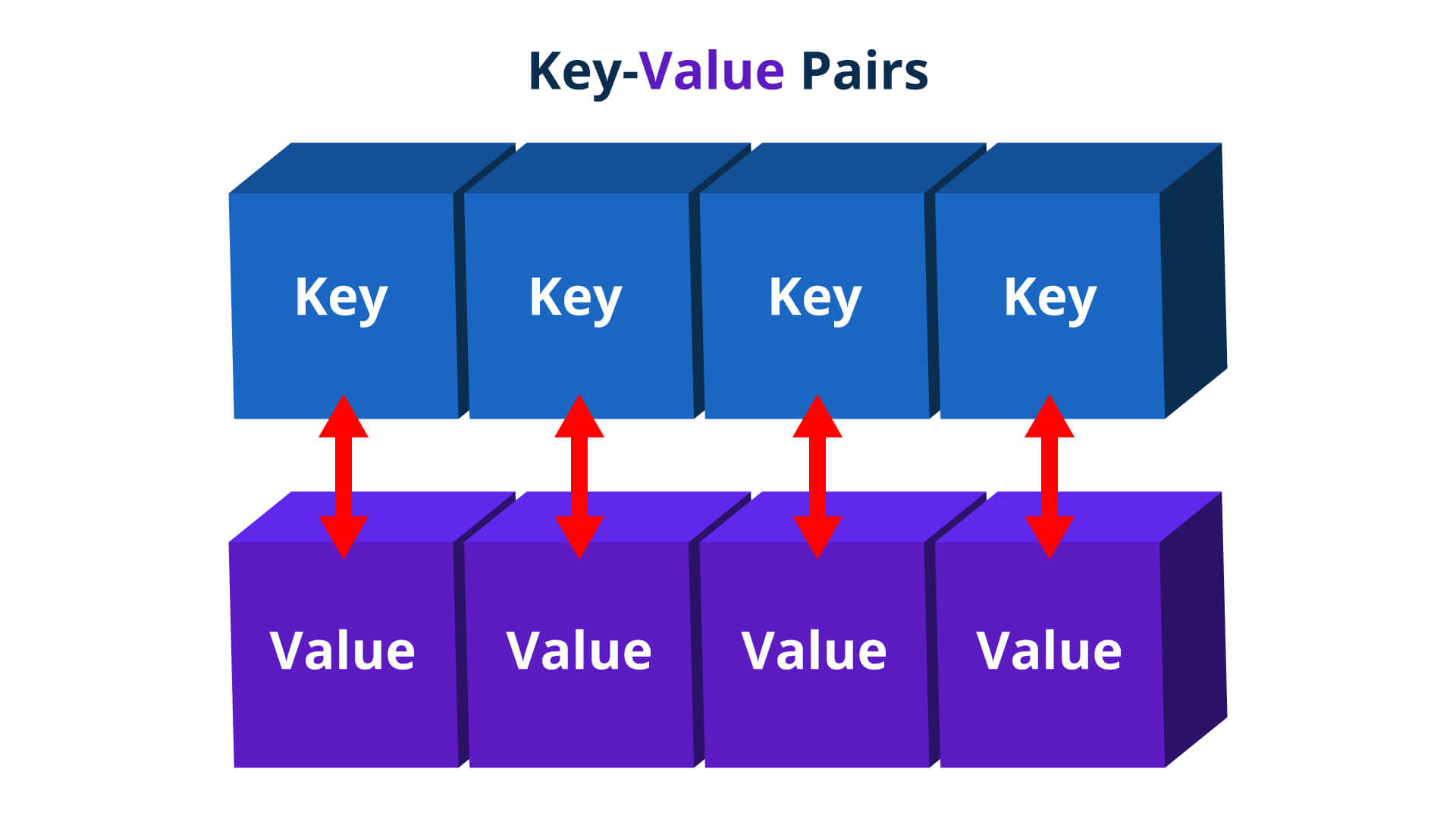
HashMap Example
How elements are stored:
Key-Value Pairs: Each element in a HashMap is stored as a key-value pair. The key is unique, and it maps to a specific value.
Fast Access: HashMap provides O(1) time complexity for basic operations like get() and put(), making it very efficient.
Null Keys and Values: HashMap allows one null key and multiple null values.
Use Cases HashMaps are versatile and can be used in various scenarios:
Caching: Storing frequently accessed data to improve performance.
Database Indexing: Quickly locating records in a database.
Configuration Management: Storing configuration settings as key-value pairs.
4.Binary Tree
A binary tree is a hierarchical data structure in which each node has at most two children, referred to as the left child and the right child1. This structure is fundamental in computer science and is used to represent hierarchical relationships between elements.
Binary Trees are a perfect candidate to use recursion.
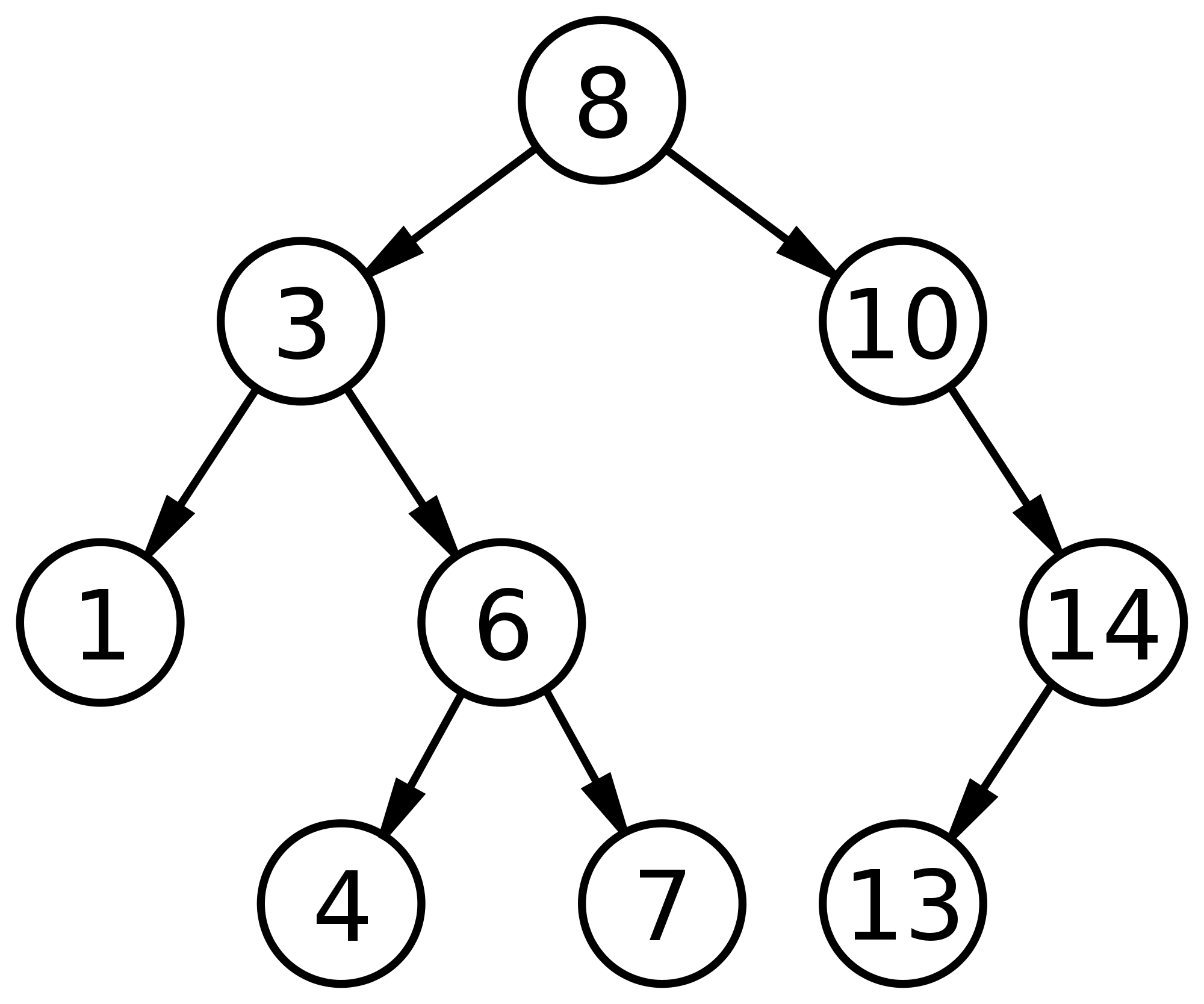
Binary Tree Example
When Binary Trees Are Used
Binary trees are used in various applications due to their efficient data organization and manipulation capabilities. Some common use cases include:
Binary Search Trees (BSTs): These are used for efficient searching, insertion, and deletion operations. BSTs maintain a sorted order of elements, which allows for quick lookup1.
Heaps: Binary heaps are used in priority queues, which are essential in algorithms like Dijkstra’s shortest path1.
- Database indexing
- Sorting Algorithms
- Decision Trees
Important concepts
- Tree Traversals:
- Preorder Traversal
- Inorder Traversal
- Postorder Traversal
Note Will cover these topics in later articles.
SubTopic:
Binary Search Tree
A Binary Search Tree (BST) is a type of binary tree where each node has a maximum of two children, and the nodes are arranged in a specific order. For any given node, all the values in its left subtree are less than the node’s value, and all the values in its right subtree are greater.
- Inorder Traversal of a BST is always a sorted elements.
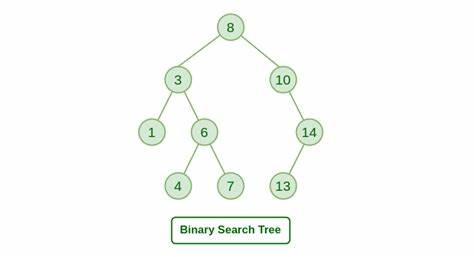
Binary Search Tree Example
5. Heaps
Heaps are a type of binary tree that satisfy the heap property, which states that for any given node (i), the value of (i) is greater than or equal to (in a max-heap) or less than or equal to (in a min-heap) the values of its children. This property makes heaps particularly useful for implementing priority queues.
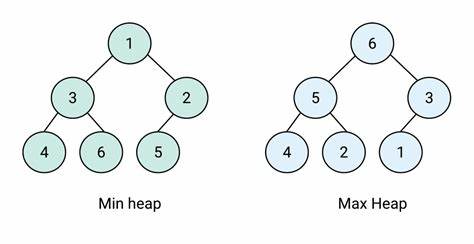
Heaps Example
Types of Heaps: There are two main types of heaps:
Max-Heap: In a max-heap, the value of each node is greater than or equal to the values of its children. The largest value is at the root.
Min-Heap: In a min-heap, the value of each node is less than or equal to the values of its children. The smallest value is at the root.
Applications of Heaps: Heaps are used in various applications, including:
Priority Queues: Heaps are often used to implement priority queues, where elements are processed based on their priority.
Heap Sort: A comparison-based sorting algorithm that uses a heap to sort elements.
Graph Algorithms: Heaps are used in algorithms like Dijkstra’s shortest path and Prim’s minimum spanning tree.
Congratulations!!, you have read the article. Remember, every line of code you write brings you one step closer to mastering the art of programming. See you in the next article. Till then, Keep learning and keep exploring.
-- From Kusuma