Understanding OOP Concepts with Real-Life Examples
1. Class and Objects
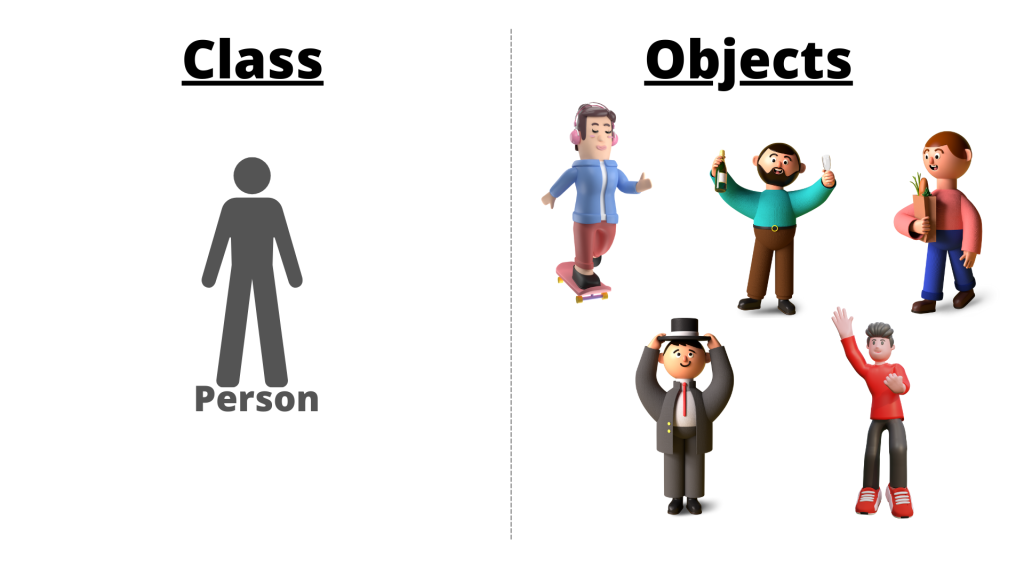
Classes and Objects
In Object-Oriented Programming (OOP), Class and Objects are the foundation of the paradigm. A class serves as a blueprint for creating objects, which are instances of the class.
Definition:
- Class: A blueprint that defines the attributes (properties) and behaviors (methods) that the objects created from the class will have.
- Object: An instance of a class. It represents a real-world entity and contains actual data.
Real-life Example:
Consider a Car as a class. The class will define attributes like the brand, color, and speed of a car, and behaviors like driving and stopping. A specific car, like a red Tesla Model 3, is an object of the Car class with its unique characteristics.
Code Examples:
#include <iostream>
using namespace std;
class Car {
public:
string brand;
string color;
void drive() {
cout << "Car is driving" << endl;
}
};
int main() {
Car car1;
car1.brand = "Tesla";
car1.color = "Red";
car1.drive();
return 0;
}
In the above C++ example, the Car class has attributes brand and color, and a method drive(). The car1 object is an instance of the Car class, representing a red Tesla.
In the above Java version, the Car class and its object car1 work similarly to the C++ example. The method drive() is called by the car1 object to display that the car is driving.
In Python, the Car class has an init constructor method that initializes the brand and color attributes. The car1 object is created with specific values, and the drive() method is called to simulate the car driving.
2. Polymorphism
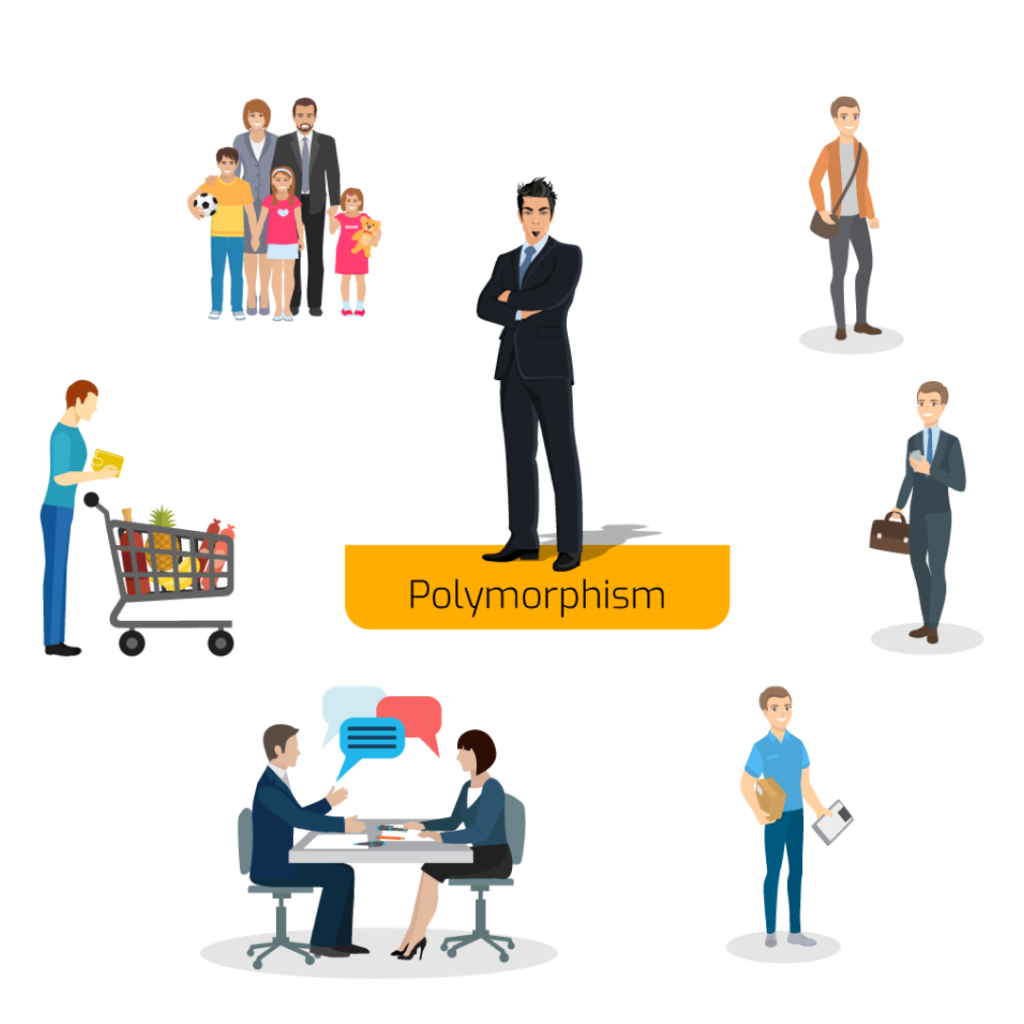
Polymorphism
Polymorphism is one of the core concepts of Object-Oriented Programming (OOP). It allows methods or objects to take on multiple forms, meaning the same method can behave differently depending on the context or the object invoking it.
Definition:
- Polymorphism: It allows one interface to be used for a general class of actions. The specific action is determined by the exact nature of the situation.
Real-life Example:
Consider a person who can take on different roles based on the context. For instance, a person may be a parent at home, an employee at work, and a customer while shopping. Though the person is the same, their behavior varies depending on the role they are playing.
Types of Polymorphism:
- Compile-time Polymorphism (Method Overloading): The function or method behavior is decided at compile time, depending on the parameters passed.
- Run-time Polymorphism (Method Overriding): The method behavior is decided at runtime, depending on the object that calls the method.
Code Examples:
#include <iostream>
using namespace std;
class Animal {
public:
virtual void sound() {
cout << "Some generic animal sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark" << endl;
}
};
int main() {
Animal* animalPtr = new Dog();
animalPtr->sound(); // Outputs "Bark"
delete animalPtr;
return 0;
}
In this C++ example, we use run-time polymorphism where the sound() method is overridden in the Dog class. The sound() method behaves differently based on the object calling it (in this case, a Dog object).
In the Java version, the sound() method in the Dog class overrides the method from the Animal class. At runtime, the correct version of sound() is called depending on the object (Dog in this case).
In Python, polymorphism is demonstrated with the sound() method overridden in the Dog class. The method behaves differently depending on whether it's called on an Animal or a Dog object.
3. Encapsulation
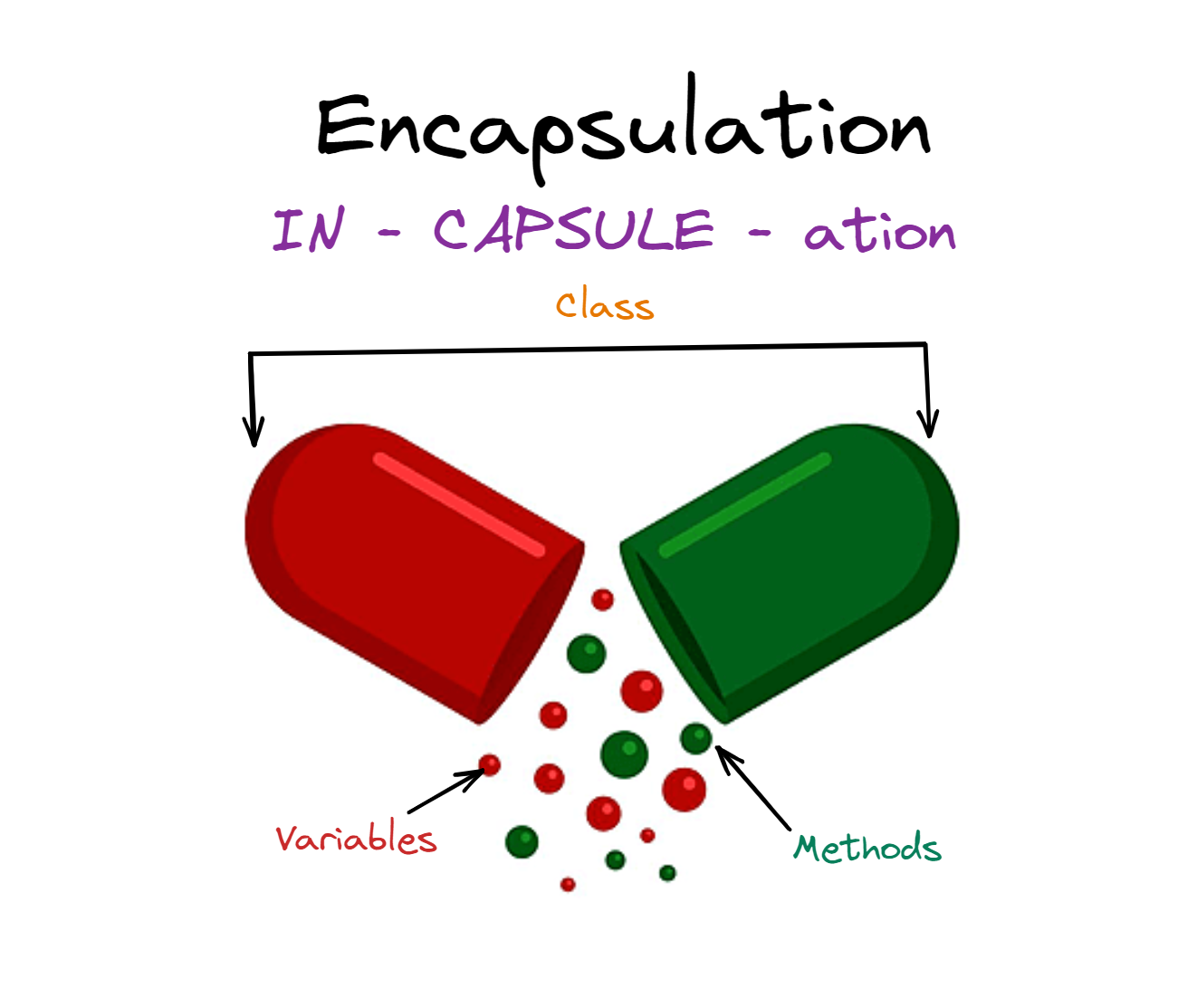
Encapsulation
Ever heard the phrase “keeping things under wraps”? Well, that’s exactly what Encapsulation is all about. It’s like wrapping up data and the methods that operate on that data into a neat little package — your class. It keeps the internal workings of an object hidden from the outside world.
Definition:
- Encapsulation: The practice of keeping an object’s data (variables) private and providing controlled access to that data through public methods. In short, it keeps the details hidden, revealing only what’s necessary.
Real-life Example:
Imagine your smartphone. You interact with it through a nice user interface (touchscreen, apps, etc.), but all the complex internal workings (wiring, code, circuitry) are hidden from you. You don’t need to know how the phone processes information, as long as it works when you tap on an app. That’s encapsulation!
Code Examples:
#include <iostream>
using namespace std;
class BankAccount {
private:
double balance; // Private variable, hidden from outside access
public:
BankAccount(double initialBalance) {
balance = initialBalance;
}
void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
double getBalance() {
return balance; // Controlled access to the private balance
}
};
int main() {
BankAccount account(1000.0);
account.deposit(500.0);
cout << "Current balance: $" << account.getBalance() << endl;
return 0;
}
In this C++ example, the balance is a private member of BankAccount. It can only be accessed through the public methods like getBalance() and deposit(). No one can mess with your balance directly (thank goodness!).
Java’s version works just like C++, where balance is hidden away and only accessed via methods. You can’t touch it directly — you gotta follow the rules 📜.
In Python, encapsulation is achieved using double underscores (__) to make balance private. The outside world can only access it through the get_balance() method — we’re keeping things tightly sealed 🔒.
4. Inheritance
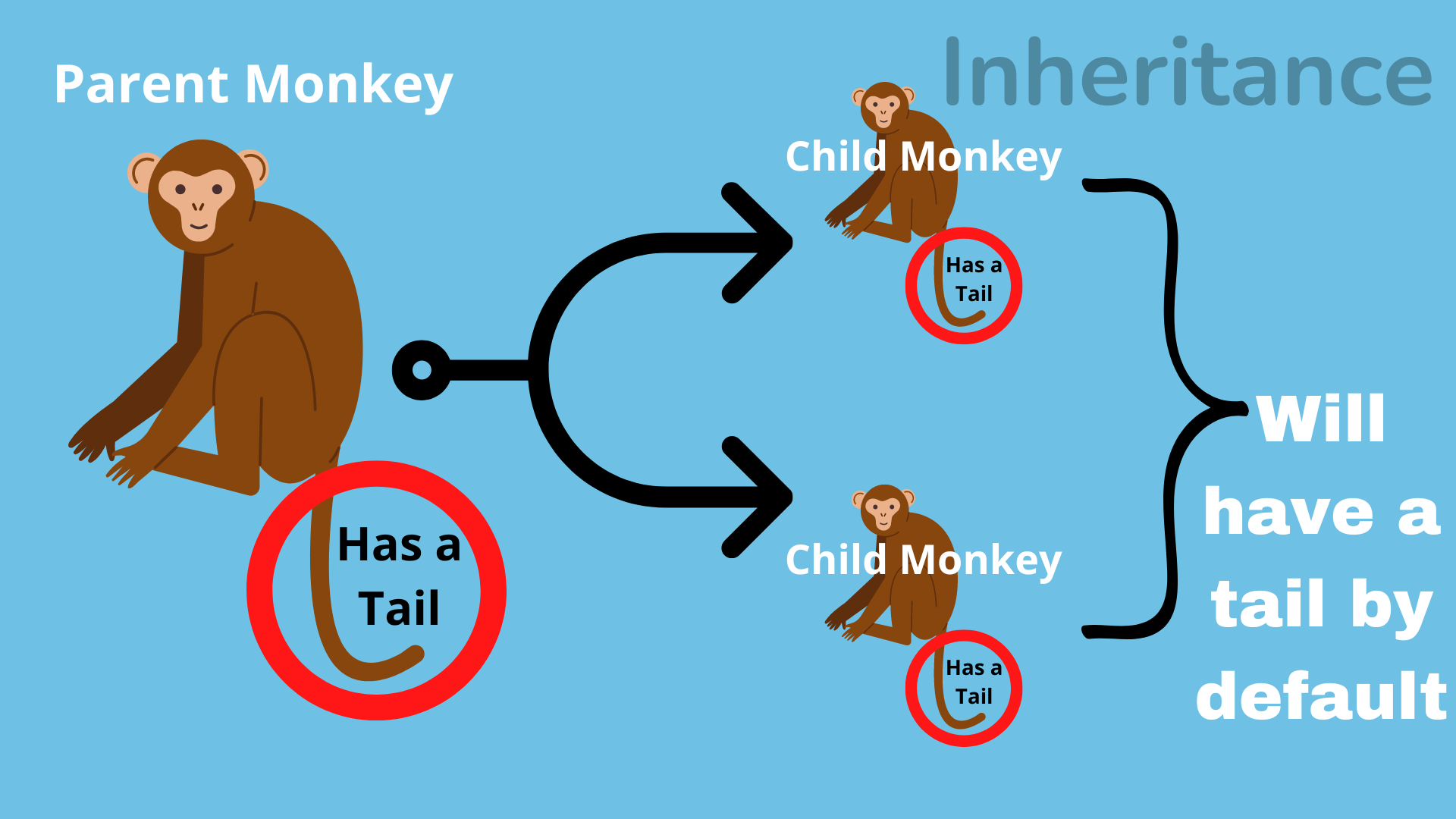
Inheritance
Inheritance is like family traits passed down from one generation to the next. In Object-Oriented Programming (OOP), it allows a new class (child class) to inherit properties and behaviors (methods) from an existing class (parent class). This promotes code reuse and establishes a hierarchical relationship between classes.
Definition:
- Inheritance: The mechanism in OOP that allows one class to inherit the attributes and methods of another class.
Real-life Example:
Think of a Vehicle class. From this class, you can create specific vehicle types like Car, Truck, and Bike. Each of these specific vehicle types inherits common properties (like wheels, speed) and behaviors (like start, stop) from the Vehicle class, but can also have their unique features (like number of doors for a Car).
Types of Inheritance:
- Single Inheritance: A child class inherits from one parent class.
- Multiple Inheritance: A child class inherits from multiple parent classes.
- Multilevel Inheritance: A class derives from a parent class, which in turn derives from another class.
- Hierarchical Inheritance: Multiple classes inherit from the same parent class.
- Hybrid Inheritance: A combination of two or more types of inheritance.
Single Inheritance:
#include <iostream>
using namespace std;
class Vehicle {
public:
void start() {
cout << "Vehicle is starting" << endl;
}
};
class Car : public Vehicle { // Car inherits from Vehicle
public:
void honk() {
cout << "Car is honking" << endl;
}
};
int main() {
Car myCar;
myCar.start(); // Inherited method
myCar.honk(); // Car-specific method
return 0;
}
In this C++ example, the Car class inherits from the Vehicle class, allowing it to use the start() method while having its own honk() method.
In the Java example, the Car class uses the extends keyword to inherit from the Vehicle class, gaining access to its methods.
In Python, the Car class inherits from the Vehicle class, allowing it to access the start() method while defining its own honk() method.
Multiple Inheritance:
#include <iostream>
using namespace std;
class Camera {
public:
void takePhoto() {
cout << "Taking a photo" << endl;
}
};
class Computer {
public:
void runProgram() {
cout << "Running a program" << endl;
}
};
class Smartphone : public Camera, public Computer { // Multiple inheritance
public:
void call() {
cout << "Making a call" << endl;
}
};
int main() {
Smartphone myPhone;
myPhone.takePhoto(); // Inherited from Camera
myPhone.runProgram(); // Inherited from Computer
myPhone.call(); // Own method
return 0;
}
In this C++ example, the Smartphone class inherits from both Camera and Computer, gaining access to their methods.
In the Java example, the Smartphone class implements two interfaces, Camera and Computer, effectively achieving multiple inheritance without class ambiguity.
In Python, the Smartphone class inherits from both Camera and Computer, allowing access to their methods directly.
Hierarchical Inheritance:
#include <iostream>
using namespace std;
class Animal {
public:
void eat() {
cout << "Eating" << endl;
}
};
class Dog : public Animal { // Dog inherits from Animal
public:
void bark() {
cout << "Barking" << endl;
}
};
class Cat : public Animal { // Cat inherits from Animal
public:
void meow() {
cout << "Meowing" << endl;
}
};
int main() {
Dog myDog;
Cat myCat;
myDog.eat(); // Inherited from Animal
myDog.bark(); // Own method
myCat.eat(); // Inherited from Animal
myCat.meow(); // Own method
return 0;
}
In this example, both Dog and Cat classes inherit from the Animal class. The Animal class provides a common method eat(), which is shared by both subclasses. Each subclass (Dog and Cat) has its own unique method (bark() and meow() respectively).
Similar to the C++ example, Dog and Cat classes extend the Animal class. Both subclasses inherit the eat() method from Animal, but also define their own specific methods (bark() for Dog and meow() for Cat), demonstrating hierarchical inheritance.
In the Python version, Dog and Cat inherit from the Animal class. The Animal class provides a shared eat() method, while each subclass defines its own behavior (bark() in Dog and meow() in Cat), illustrating hierarchical inheritance.
Hybrid Inheritance:
#include <iostream>
using namespace std;
class Animal {
public:
void eat() {
cout << "Eating" << endl;
}
};
class Mammal : public Animal {
public:
void walk() {
cout << "Walking" << endl;
}
};
class Bird : public Animal {
public:
void fly() {
cout << "Flying" << endl;
}
};
class Bat : public Mammal, public Bird { // Multiple inheritance
public:
void echoLocate() {
cout << "Using echolocation" << endl;
}
};
int main() {
Bat myBat;
myBat.eat(); // Inherited from Animal
myBat.walk(); // Inherited from Mammal
myBat.fly(); // Inherited from Bird
myBat.echoLocate(); // Bat's own method
return 0;
}
Data Abstraction
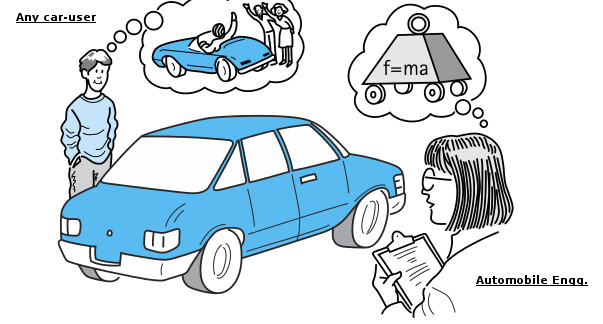
Data Abstraction
Data Abstraction refers to the concept of hiding the complex implementation details from the user and only exposing the essential features or functionalities. It allows focusing on what an object does instead of how it does it.
Definition:
- Data Abstraction: The process of providing only necessary details to the outside world while keeping the internal implementation hidden. It is a key concept of Object-Oriented Programming (OOP) used to reduce complexity and increase efficiency.
Real-life Example:
Consider a TV Remote. When you press the power button, the TV turns on. You don’t need to know how the electrical circuit inside the TV works; you just care about the functionality of turning the TV on or off. This is data abstraction in real life: hiding the internal complexities and exposing only the relevant operations.
Code Examples:
#include <iostream>
using namespace std;
class RemoteControl {
private:
void turnOnCircuit() { // Hidden implementation
cout << "Turning on the TV's circuit" << endl;
}
public:
void pressPowerButton() { // Exposed functionality
turnOnCircuit();
cout << "TV is now ON" << endl;
}
};
int main() {
RemoteControl remote;
remote.pressPowerButton(); // User only interacts with the power button
return 0;
}
In this C++ example, the internal function turnOnCircuit() is hidden from the user, and the user interacts only with the pressPowerButton() function.
In this Java example, the turnOnCircuit() method is private and hidden, while the public method pressPowerButton() is exposed for the user to interact with.
In Python, the method __turn_on_circuit() is marked as private using double underscores, and only press_power_button() is exposed to the user.
Conclusion: Wrapping Up OOP Concepts! 🎉
Object-Oriented Programming (OOP) is the foundation of modern software development. By understanding the pillars of OOP—Classes, Objects, Polymorphism, Encapsulation, Inheritance, and Data Abstraction—you’re now equipped with powerful tools to build scalable, maintainable, and flexible applications. 🚀
Key Takeaways:
- Classes and Objects form the blueprint and instances of your programs.
- Polymorphism allows for flexibility in method usage based on the context.
- Encapsulation safeguards data and provides controlled access.
- Inheritance promotes code reuse, enhancing efficiency.
- Data Abstraction hides the complexity and shows only essential features to users.
By mastering these concepts, you’re not only writing better code, but you're also thinking in a way that will make future software challenges easier to tackle! 💡
Keep coding, keep learning, and see you in the next article! 👨💻👩💻 - Nehal Fathema